In the Previous article we have discussed how we can get the API keys and secret from Angel one Demat Account and How we can auto generate TOTP using python for angel one smart API
In this Article we will learn how to get Historical Data from Angel One smart API using python for this you have to complete previous articles. After completed those two articles our Python file will look like below.
from smartapi import SmartConnect
import os
from pyotp import TOTP
from dotenv import load_dotenv
import urllib
import json
import pandas as pd
import datetime as dt
import time
load_dotenv()
# Get TOTP Token From the ENV file
AngelTotptoken = os.environ.get("AngelTtoken")
#Get API Key From ENV File
AngelApiKey = os.environ.get("AngelAPiKey")
#Get Client ID From ENV File
AngelClientId = os.environ.get("AngelClientId")
#GET Client Password or MPIN
AngelPassword = os.environ.get("AngelPassword");
myTotp = TOTP(AngelTotptoken).now()
Now we have to generate Angel one session to do any kind of activity like get historical data or placing orders etc , paste following code to your python file to generate Angel one session.
obj=SmartConnect(api_key=AngelApiKey)
data = obj.generateSession(AngelClientId,AngelPassword,myTotp)
How to Get Historical Data From Angel One smart API using getCandleData function.
We have to pass some parameters to getCandleData parameters to get historical data which are following .
- exchange
- symboltoken
- interval
- fromdate
- todate
(1) Exchange: we will pas NSE for Equity segment
(2) Symboltoken: Angel one provides a list of tradable instruments in json format so we have to find symboltoken from this json file for any equity stock.
(3) Interval: we have to tell angel one api that for which time frame we are trying to get historical data, Angel one provides data for the following time frame.
- ONE_MINUTE
- THREE_MINUTE
- FIVE_MINUTE
- TEN_MINUTE
- FIFTEEN_MINUTE
- THIRTY_MINUTE
- ONE_HOUR
- ONE_DAY
Example: If we pass ONE_MINUTE as an interval angel one will provide OHLC data for every minute.
(4) From Date: will pass datetime in yyyy-MM-dd hh:mm format.
(5) TO Date: Will pass datetime in yyyy-MM-dd hh:mm format.
Now we need the symbol token, Angel one provides all tradable instruments list in JSON format, you have to hit this URL Tradable Instruments List.
To get json data from this url we are using urllib & json python library. Please import the following libraries and paste the code in your python file.
instruments_url = "https://margincalculator.angelbroking.com/OpenAPI_File/files/OpenAPIScripMaster.json"
response = urllib.request.urlopen(instruments_url);
instruments_list = json.loads(response.read())
if you print the instruments_list variable output will look like below image I am using spyder3 IDE you can you any other which is suitable to you.

Let’s define one separate function for getting a symbol token for a given equity ticker name, paste following code in your python file.
# Function For get token from the ticker name
def get_token_from_ticker(ticker,instruments_list,exchange= "NSE" ):
for instrument in instruments_list:
if(instrument["name"] == ticker and instrument["exch_seg"] == exchange and instrument['symbol'].split("-")[-1] == "EQ" ):
return instrument["token"]
We can pass any equity stock name in the ticker let’s take one example.
infy_token = get_token_from_ticker("INFY",instruments_list)
print(infy_token)
# output will look like 1594 which is the token for infosys equity
Now we have everything which must for getting Historical Data for Indian Stock Market From Angel One Demat let’s try to get the data of infosys for 15 minute time frame . past following code in your python file.
First of all we will define one function for getting the historical data for any token so we can use it infinite time.
def get_hist_data(duration, interval,token, exchange = "NSE"):
st_date = st_date = dt.date.today() - dt.timedelta(duration)
end_date = dt.date.today()
params = {
"exchange": exchange,
"symboltoken": token,
"interval": interval,
"fromdate": (st_date).strftime("%Y-%m-%d %H:%M"),
"todate": end_date.strftime("%Y-%m-%d %H:%M")
}
hist_data = obj.getCandleData(params)
return hist_data
We will pass duration as 10 days, interval as FIFTEEN_MINUTE and token as infy_token in which we stored the token for infosys equity. means we are trying to get the 10 days of Historical data of infosys for 15 min time frame the function return us 15 min OHLC data for infosy.
get_hist_data(10, "FIFTEEN_MINUTE",infy_token)
out put will look like below.
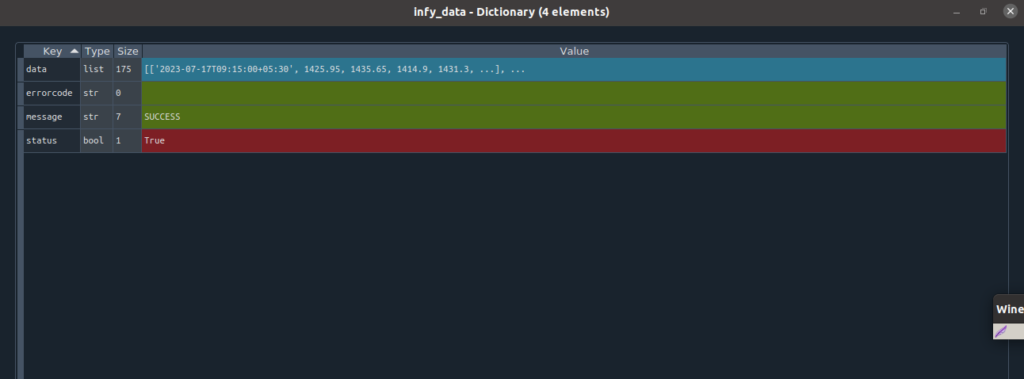
Angel return data in dictionary format for better readability we will convert it on Data Frame using Pandas so we have to do little change in our get_hist_data function
Updated get_hist_data function
def get_hist_data(duration, interval,token, exchange = "NSE"):
st_date = st_date = dt.date.today() - dt.timedelta(duration)
end_date = dt.date.today()
params = {
"exchange": exchange,
"symboltoken": token,
"interval": interval,
"fromdate": (st_date).strftime("%Y-%m-%d %H:%M"),
"todate": end_date.strftime("%Y-%m-%d %H:%M")
}
hist_data = obj.getCandleData(params)
df_data =pd.DataFrame(hist_data['data'],
columns = ["date","open","high","low","close","volumn"])
df_data.set_index("date",inplace=True)
df_data.index= pd.to_datetime(df_data.index)
df_data.index = df_data.index.tz_localize(None)
return df_data
We have converted the python dictionary to Data frame and set date to index and remove the unnecessary timezone related string from the Date index , now we have flowing output which is neat and clean and easy to read.
10 days of Open , High , Low , Close and Volume Data For Infosys Equity.
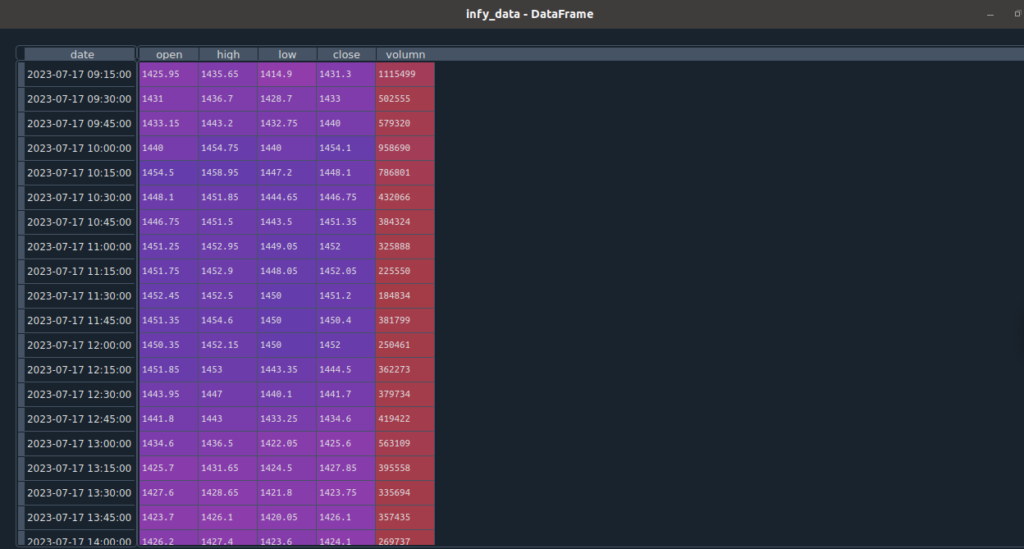
if you like my efforts and you really got some useful information from this Article please share it with you friends and family. see you in next Article with some more interesting topics. Jay hind
Thumbnail Image Credit Image by pikisuperstar on Freepik
Subscribe to our email newsletter to get the latest posts delivered right to your email.
Comments